데브리입니다. 이제 가능하면 새로운 프로젝트를 만들 때 자바스크립트가 아닌 타입스크립트를 쓰고 싶은데 맛보기로 무료 강의를 찾아보다가 노마드코터 니코쌤 무료 강의가 있어서 듣게 되었어요. 그야말로 맛보기로 들었고, 뒤에 어려운 부분은 흘려들었지만 타입스크립트가 처음이신 분들은 한번쯤 들어보셔도 좋을 것 같네요.
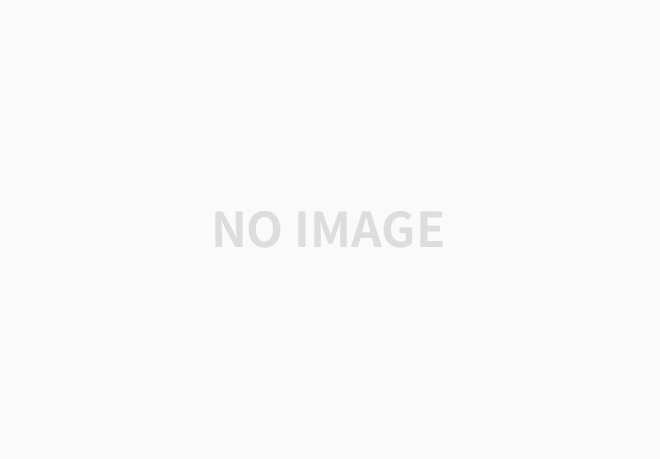
아직 어떤 type이 될지 모를 때
let a: unknown;
if(typeof a === "number"){
let b = a + 1
}
if(typeof a === "string"){
let b = a.toUpperCase();
}
void
아무것도 return하지 않는 function
function hello(){
console.log('x')
마우스오버하면 function hello():void 라고 나오고 Typescript가 자동으로 인식하기 때문에 따로 void를 쓸 필요는 없음.
never
function이 절대 return하지 않을 때 발생함. 거의 쓸 일이 없겠지만 기억해두면 좋음.
function hello():never{
throw new Error("xxx");
}
throw new를 사용할 경우 return하지 않고 오류를 발생시킬 수 있음.
Call Signatures
type Add = (a:number, b:number) => number;
const add:Add = (a,b) => a + b
Overloading
하나의 function이 여러개의 다른 call signatures를 가지고 있을 때 발생.
type Push = {
(path:string):void
(config: Config):void
}
const push:Push = (config) => {
if(typeof config === "string"){console.log(config)}
else {
console.log(config.path)
}
}
Polymorphism
여러 형태를 가진 하나의 function
type SuperPrint = {
<TypePlaceholder>(arr: TypePlaceholder[]):void
}
const superPrint: SuperPrint = (arr) => {
arr.forEach(i => consoloe.log(i))
}
superPrint([1, 2, 3, 4])
superPrint([true, false, true])
superPrint(["a", "b", "c"])
superPrint([1, 2, true, false, "hello"])
concrete type이 아닌 generic type을 받도록 함. 어떤 type이 들어갈지 미리 알 수 없을 때 쓸 수 있어서 유용함.
<TypePlaceholder> 대신 아무거나 임의로 넣을 수 있음. <T>,<V> 등이 보편적으로 많이 쓰임.
type SuperPrint = <T>(a: T[]) => T
const superPrint: SuperPrint = (a) => a[0]
superPrint([1, 2, 3, 4])
superPrint([true, false, true])
superPrint(["a", "b", "c"])
superPrint([1, 2, true, false, "hello"])
혹은
function superPrint<T>(a: T[]){
return a[0]
}
하지만 직접 라이브러리를 만들지 않는 이상, React나 Next.js에서는 generic을 사용하게는 되겠지만 직접 작성할 일은 없음.
"use strict"
class Player {
constructor(firstName, lastName){
this.firstName = firstName;
this.lastName = lastName;
}
}
class Player {
constructor(
private firstName: string,
private lastName: string
public nickName: string
) {}
}
abstract class User {
constructor(
protected firstName: string,
protected lastName: string,
protected nickname: string
){}
abstract getNickName():void
getFullName(){
return `${this.firstName} ${this.lastName}`
}
}
type vs interface
type: 다양한 목적으로 사용될 수 있음. type 키워드를 사용해 object를 정해줄 수 있고 특정값이나 tpe alias를 만들 수 있음
interface: 단 한가지 목적만을 가짐. 오직 object shape을 타입스크립트에게 설명해주기 위해 사용되는 키워드.
interface는 원하는 method와 property를 class가 가지도록 force할 수 있음.
interface는 자바스크립트로 컴파일되지 않음. abstract class와 비슷한 보호를 제공하지만 자바스크립트 파일에서 보이지 않음.
* type과 interface는 비슷하지만 차이가 있음.
type: cannot be re-opened to add new properties
interface: always extendable
interface User {
firstName:string,
lastName:string,
sayHi(name:string):string
fullName():string
}
class Player implements User {
constructor(
public firstName:string,
public lastName:string
){}
fullName(){
return `${this.firstName} ${this.lastName}`
}
sayHi(name:string){
return `Hello ${name}, My name is ${this.fullName()}`
}
Definitely Typed / types
오픈소스 프로젝트 for typescript로 프로젝트를 만들 때 필요한 패키지를 여기서 찾을 수 있음.
nodejs type definitions for typescript
코드를 그대로 복사해도 되지만 terminal에서 설치할 수도 있음
npm i -D @types/node
JSDoc
자바스크립트로 만들어진 기존 프로젝트가 있고, 새 코드는 타입스크립트로 작성하면 되지만 기존의 자바스크립 파일도 타입스크립트처럼 protect 받고 싶을 때 // @ts-check 코멘트만 작성하면 됨.
추가 공부
The TypeScript Handbook
* 함께 읽으면 좋은 글
2021.09.20 - [웹개발/혼자하는 개발 공부] - 타입스크립트 TypeScript 란 무엇이며 왜 써야하는가?
타입스크립트 TypeScript 란 무엇이며 왜 써야하는가?
TypeScript is a variant of JavaScript. It is useful when it comes to large-sized codebase and distributed teams working on the same project. 자바스크립트는 dynamically typed 언어라, 오직 런타임에서만 에러를 확인할 수 있다. 이
devlee.tistory.com
'웹개발 > 혼자하는 개발 공부' 카테고리의 다른 글
React vs Angular vs Vue (0) | 2023.07.21 |
---|---|
node 낮은 버전으로 downgrade하는 법 (create-react-app 오류 해결) (0) | 2023.06.13 |
2023년 기준 Responsive Design 모바일 & 테블릿 사이즈 (Media Query) (0) | 2023.05.09 |
Angular 기초 (0) | 2023.05.01 |
프론트엔드 실력 향상하기 + 인터뷰 준비 (2) | 2023.04.22 |